Hmmm... Very very interesting. If accuracy and speed somehow improves, SURF can change the direction of AR.
Videos:
openFrameworks & OpenCV SURF from postspectacular on Vimeo.
Pages - Menu
▼
Saturday, February 20, 2010
Wednesday, February 10, 2010
OpenCV + cvblobslib Demo program
Here is a Sample application to display an image.
Download the Project folder and the source code.
Download the Project folder and the source code.
//______________________________________________________________________________________ // OpenCV Blobs detection program // Author: Bharath Prabhuswamy //______________________________________________________________________________________ //______________________________________________________________________________________ #include <stdio.h> #include "cv.h" #include "highgui.h" #include "BlobResult.h" int main() { CvCapture *capture = 0; IplImage *img = 0; IplImage *gray_img = 0; IplImage *thres_img = 0; IplImage *blobs_img = 0; int key = 0; /* Initialize the webcam */ capture = cvCaptureFromCAM( 0 ); /* Always check if the program can find a device */ if ( !capture ) return -1; CBlobResult blobs; CBlob *currentBlob; int frame_count= 0; int i; /* create a window for the video */ //cvNamedWindow( "Webcam",CV_WINDOW_AUTOSIZE); cvNamedWindow( "Blobs",CV_WINDOW_AUTOSIZE); /* Create required images once */ if( frame_count == 0 ) { /* Obtain a frame from the device */ img = cvQueryFrame( capture ); /* Always check if the device returns a frame */ if( !img ) return -1; gray_img = cvCreateImage( cvGetSize(img), img->depth, 1); thres_img = cvCreateImage( cvGetSize(img), img->depth, 1); blobs_img = cvCreateImage( cvGetSize(img), img->depth, 3); } while((char) key != 27 ) { /* Obtain a frame from the device */ img = cvQueryFrame( capture ); /* Always check if the device returns a frame */ if( !img ) return -1; frame_count = frame_count + 1; /* Flip image once, after blob processing it is flipped back */ cvFlip(img,img,NULL); /* Convert image from Color to grayscale and then to binary (thresholded at 200) */ cvCvtColor(img,gray_img,CV_RGB2GRAY); cvThreshold(gray_img,thres_img,200,255,CV_THRESH_BINARY); /* Find Blobs that are White, Hence 'uchar backgroundColor = 0' (Black) */ blobs = CBlobResult(thres_img, NULL,0); /* Remove blobs if it does not cover minimum area specified below */ blobs.Filter( blobs, B_EXCLUDE, CBlobGetArea(),B_LESS,5,50); /* Number of blobs */ int j = blobs.GetNumBlobs(); printf("%d\n",j); /* Color the blobs one after the other */ for (i = 0; i < blobs.GetNumBlobs(); i++ ) { currentBlob = blobs.GetBlob(i); currentBlob->FillBlob( blobs_img, CV_RGB(255,0,0)); } //cvShowImage( "Webcam",gray_img); cvShowImage( "Blobs",blobs_img); /* Clear image for next iteration */ cvZero(blobs_img); /* Quit execution if 'ESC' key is pressed */ key = cvWaitKey(1); } /* Clean up memory */ //cvDestroyWindow( "Webcam" ); cvDestroyWindow( "Blobs" ); cvReleaseCapture( &capture ); return 0; }
cvblobslib with OpenCV : Installation
Here are the details for installing cvblobslib. If you want save this post for reading it later, download the PDF from the link below.
Download cvblobslib Howto [PDF & Snapshots]
cvblobslib
There are numerous blob tracking libraries of which cvblobslib is discussed below:
Download the source from the link below:
cvblobslib_OpenCV_v8_3.zip Latest release: 8.3
For documentation, download the attachment :
cvblobslib_v5_doc.zip
Extract .zip file into a folder.
cvblobslib – Visual Studio
For details refer:
It is necessary that cvblobslib.lib (library file) is generated in order to make use of the blob methods. The following steps are followed in order to generate this library file from the files just extracted.
Open the project and set the following parameters:
1. Open Project -> Properties ->C/C++ -> Precompiled Headers and select Not use precompiled headers.
- Open Project -> Properties -> C/C++-> Code Generation. Select Run-time library and set it to Multi-threaded Debug DLL (/MDd) (debug version) and/or to Multi-threaded DLL (/MD) (release version).
- Open Project -> Properties -> Configuration Properties -> General. Select Use of MFC and set to Use MFC in a shared DLL.
Images:









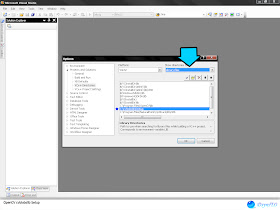



OpenCV : Accessing the Webcam
Here is a Sample program to access the webcam.
Download the Project folder and the source code.
Download the Project folder and the source code.
//______________________________________________________________________________________ // OpenCV Webcam access // Author: Bharath Prabhuswamy //______________________________________________________________________________________ //______________________________________________________________________________________ #include "cv.h" #include "highgui.h" #include <stdio.h> int main() { CvCapture *capture = 0; IplImage *img = 0; char key = 0; /* Initialize the webcam */ capture = cvCaptureFromCAM( 0 ); /* Always check if the program can find a device */ if ( !capture ) return -1; cvNamedWindow( "Webcam",CV_WINDOW_AUTOSIZE); while((char) key != 27 ) { /* Obtain a frame from the device */ img = cvQueryFrame( capture ); /* Always check if the device returns a frame */ if( !img ) return -1; cvShowImage( "Webcam",img); /* Quit execution if 'ESC' key is pressed */ key = cvWaitKey(1); } /* Clean up memory */ cvDestroyWindow( "Webcam" ); cvReleaseCapture( &capture ); return 0; }
OpenCV : Displaying an Image
Here is a Sample application to display an image.
Download the Project folder and the source code.
Download the Project folder and the source code.
//______________________________________________________________________________________ // OpenCV Demo Program // Author: Bharath Prabhuswamy //______________________________________________________________________________________ //______________________________________________________________________________________ #include "cv.h" #include "highgui.h" #include <stdio.h> int main() { cvNamedWindow( "image", CV_WINDOW_AUTOSIZE); /* Load an Image from the disk */ IplImage* img = cvLoadImage("things.jpg"); /* Always check if the program can find the file */ if( !img ) return -1; /* Display the image in the window named "image" */ cvShowImage( "image", img ); cvWaitKey(0); /* Clean up memory */ cvDestroyWindow( "image" ); cvReleaseImage( &img ); return 0; }
OpenCV: Installation
Here are the details for installing OpenCV. If you want save this post for reading it later, download the PDF from the link below.
Download OpenCV Howto [PDF & Snapshots]
Installing OpenCV 1.0
Download Executable from the link below:
The file will be present in the folders:
opencv-win
> 1.0
> OpenCV_1.0.exe 18.0 Mb
Install the the package.
Visual Studio
Global Settings:
Include the directories of the OpenCV headers and OpenCV libraries into the Visual Studio.
Before opening any projects, open Tools from the menu bar and select Options.
In the Options window select VC++ Directories. Open Show the Directories for: and select Include files.
Now add the following paths where the OpenCV include files are present.
C:\Program Files\OpenCV\cv\include
C:\Program Files\OpenCV\cxcore\include
C:\Program Files\OpenCV\otherlibs\highgui
C:\Program Files\OpenCV\cvaux\include
C:\Program Files\OpenCV\otherlibs\_graphics\include
Similarly select Source files from Show the Directories for: option. Now add the path where the source files are present.
C:\Program Files\OpenCV\cv\src
C:\Program Files\OpenCV\cxcore\src
C:\Program Files\OpenCV\cvaux\src
C:\Program Files\OpenCV\otherlibs\highgui
C:\Program Files\OpenCV\otherlibs\_graphics\src
Similarly select Library files from Show the Directories for: option & add the path where library files are present.
C:\Program Files\OpenCV\lib
Click on OK to save the changes
Now all you have to do is create a new cpp project and add the following headers to your program -
#include <cv.h>
#include <cxcore.h>
#include <highgui.h>
Project Settings:
Before building a project, Right click on the project and open Properties. Now click Linker and select Input. Type cxcore.lib cv.lib highgui.lib in to Additional Dependances.
Image:






