Open a new project and enter the code. Right click on the project and open properties. Now click Linker and select Input. Type optitrackuuid.lib in to Additional Dependances.
Important notes:
Component Object Model (COM)
It is a binary-interface. It is used to enable inter-process communication and dynamic object creation.
ATL [library/Framework]
The Active Template Library (ATL) is a set of template-based C++ classes that simplify the programming of Component Object Model (COM) objects.
Com provides a method for sharing binary code across different applications and languages.With COM, A component can be created which communicates with programs that are written in any software language. Using ATL, it is possible to add “parts” to the component easily.
Let us assume that a camera is initialized as COM object. By making use of ATL, frame count (an integer type) is defined as a member of this camera. Since the camera is a COM object, there is an interface to access camera and obtain the frame count via a C++ program or a java program alike. And the programs can access and pass these “members” to each other. Thus in COM the Components, Objects, classes and its members remain unaffected by the language used.
For more interesting Explanation:
Sample Code:
//______________________________________________________________________________________ // Marker Detection Demo Program // Author: Bharath Prabhuswamy //______________________________________________________________________________________ //______________________________________________________________________________________ #pragma once #define WIN32_LEAN_AND_MEAN #define _USE_MATH_DEFINES #include <conio.h> #include <objbase.h> #include <atlbase.h> #include "optitrack.h" #import "optitrack.tlb" int _tmain(int argc, _TCHAR* argv[]) { printf("** Marker Detection Demo Program --------------------------------------------\n"); printf("** Author: Bharath Prabhuswamy --------------------------------------------\n\n"); //Initialize COM__________________________________________________ CoInitialize(NULL); //Initialize Optitrack COM Objects________________________________ CComPtr<inpcameracollection> cameraCollection; CComPtr<inpcamera> camera; CComPtr<inpcameraframe> frame; //Create uninitialized object of the class associated CLSID_______ cameraCollection.CoCreateInstance(CLSID_NPCameraCollection); //Enumerate (Identify) Available Cameras__________________________ cameraCollection->Enum(); long cameraCount = 0; int frameCounter = 0; //Determine Available Cameras_____________________________________ cameraCollection->get_Count(&cameraCount); //Open the camera if any detected_________________________________ if(cameraCount>0) { cameraCollection->Item(0, &camera); { //Open camera 0 (i.e. first camera), and set various parameters___ cameraCollection->Item(0, &camera); //Set Greyscale Mode_____________________________________________ camera->SetOption(NP_OPTION_VIDEO_TYPE , (CComVariant) 1 ); //Set to discard every other frame out of 120 frames //(i.e. 60 Frames/Second)_________________________________________ camera->SetOption(NP_OPTION_FRAME_DECIMATION , (CComVariant) 1 ); //Set User defined Threshold_______________________________________ camera->SetOption(NP_OPTION_THRESHOLD , (CComVariant) 253); //Set User defined Intensity_______________________________________ camera->SetOption(NP_OPTION_INTENSITY , (CComVariant) 15); //Set User defined Exposure________________________________________ camera->SetOption(NP_OPTION_EXPOSURE , (CComVariant) 201); //Set User defined Ranking options_________________________________ camera->SetOption(NP_OPTION_OBJECT_MASS_WEIGHT , (CComVariant) 0); camera->SetOption(NP_OPTION_OBJECT_RATIO_WEIGHT , (CComVariant) 2); camera->SetOption(NP_OPTION_PROXIMITY_WEIGHT , (CComVariant) 0); camera->SetOption(NP_OPTION_STATIC_COUNT_WEIGHT , (CComVariant) 0); camera->SetOption(NP_OPTION_SCREEN_CENTER_WEIGHT , (CComVariant) 0); camera->SetOption(NP_OPTION_LAST_OBJECT_TRACKED_WEIGHT , (CComVariant) 10); camera->Open(); camera->Start(); { while(!_kbhit()) { camera->GetFrame(0, &frame); if(frame!=0) { //Increment Frame Counter_____________________________________ frameCounter++; //Process Every Frame_________________________________________ if((frameCounter%1==0)) { long Objcount = 0; long rank; CComVariant X, Y, Area; //Obtain Objects from frame of camera________________________ frame->get_Count(&Objcount); //Get data of all objects____________________________________ for (int i = 0; i < Objcount; i++) { CComPtr<inpobject> object; frame->Item(i,&object); object->Transform(camera); object->get_Rank(&rank); //Store data of object with first rank______________________ if(rank == 1) { object->get_X(&X); object->get_Y(&Y); object->get_Area(&Area); } } //Print the values of the object_____________________________ printf("X value is =%f/tY value is =%f/tArea is =%f\n\n",X.dblVal, Y.dblVal, Area.dblVal); } frame->Free(); frame.Release(); } } } camera->Stop(); camera->Close(); } camera.Release(); } //Clean-up COM Objects____________________________________________ cameraCollection.Release(); //Uninitialize COM________________________________________________ CoUninitialize(); printf("Application Exit.\n"); return 0; }Downloads:
Download Project folder with Source 2.89Mb
Images:
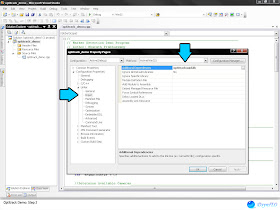